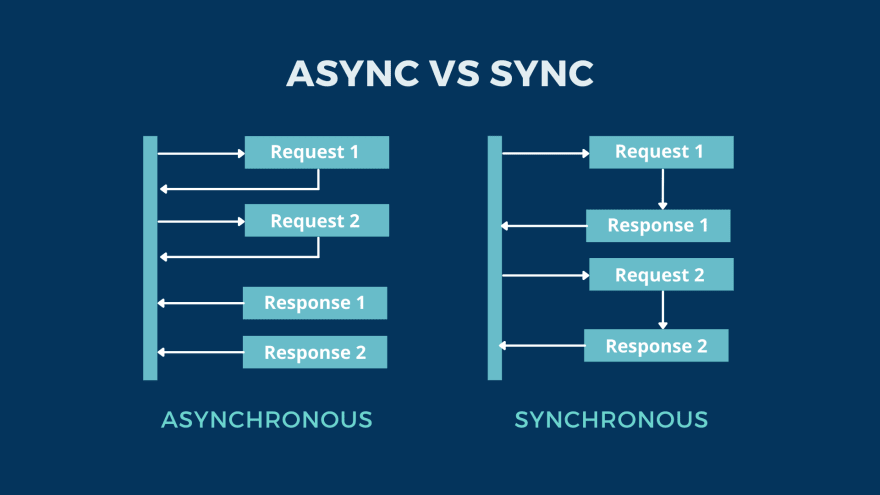
In the fast-paced world of web development, mastering asynchronous JavaScript and REST APIs is crucial for building efficient and responsive applications. Whether you’re a seasoned developer or just starting, understanding these concepts will significantly improve your ability to handle data requests and enhance user experience. This article will delve into the intricacies of async JavaScript and REST APIs, providing you with practical knowledge, tips, and best practices to elevate your coding skills.
What Is Async JavaScript and Why Is It Important?
Async JavaScript, short for asynchronous JavaScript, allows you to perform tasks without blocking the main thread of execution. This means you can execute code in the background without freezing the user interface. It's especially useful when dealing with tasks like fetching data from APIs, handling user input, or performing time-consuming operations.
The Evolution of JavaScript
JavaScript wasn’t always asynchronous. In its early days, it relied heavily on callbacks, which often led to what’s known as “callback hell”—a tangled mess of nested functions that made code difficult to read and maintain. With the introduction of Promises and, later, the async
and await
syntax, JavaScript has become more manageable and readable.
REST APIs: The Backbone of Modern Web Applications
A REST API (Representational State Transfer Application Programming Interface) is a set of rules and conventions for building and interacting with web services. It allows different software applications to communicate over HTTP using standard methods like GET, POST, PUT, and DELETE.
REST APIs are widely used for building web and mobile applications because they provide a scalable and efficient way to handle data requests. When combined with async JavaScript, they enable developers to build responsive and high-performance applications.
How Async JavaScript Works with REST APIs
Async JavaScript and REST APIs work hand-in-hand to perform data operations smoothly. By using async
and await
, you can make API requests in a non-blocking manner, allowing your application to remain responsive even while waiting for data to be fetched.
Here’s a basic example of how async JavaScript interacts with a REST API:
async function fetchData() {
try {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData();
In this example, the fetchData
function sends a request to the API and waits for the response. The await
keyword pauses the function execution until the data is received, preventing the need for complex callback functions.
Key Concepts in Async JavaScript and REST APIs
1. Promises
Promises are objects representing the eventual completion or failure of an asynchronous operation. They can have three states: pending, fulfilled, or rejected.
let promise = new Promise((resolve, reject) => {
// Async operation
setTimeout(() => resolve('Data fetched!'), 2000);
});
promise.then(result => console.log(result)); // Outputs 'Data fetched!' after 2 seconds
2. Async and Await
The async
keyword is used to define an asynchronous function, and await
pauses the execution of that function until the Promise is resolved.
async function exampleFunction() {
let result = await someAsyncOperation();
console.log(result);
}
3. Error Handling
Using try
and catch
blocks, you can handle errors gracefully in async functions.
async function getData() {
try {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
console.log(data);
} catch (error) {
console.error('Failed to fetch data:', error);
}
}
Practical Use Cases of Async JavaScript and REST APIs
1. Building Dynamic User Interfaces
Async JavaScript is ideal for building dynamic UIs where data is fetched from APIs and displayed to users without reloading the page. For example, a news website can use async functions to load articles as the user scrolls, providing a seamless reading experience.
2. Form Submissions
Using async functions, you can handle form submissions without refreshing the page, enhancing user experience. The form data is sent to the server via a POST request, and the response can be used to update the UI dynamically.
async function submitForm(event) {
event.preventDefault();
let formData = new FormData(event.target);
try {
let response = await fetch('/submit', {
method: 'POST',
body: formData
});
let result = await response.json();
console.log(result);
} catch (error) {
console.error('Form submission failed:', error);
}
}
3. Fetching and Displaying Data
Many applications rely on real-time data to function effectively. Whether it's a stock market app or a weather forecast tool, async JavaScript and REST APIs enable these applications to fetch and display the latest data without disruptions.
Common Pitfalls and How to Avoid Them
1. Handling Multiple API Requests
Making multiple API requests simultaneously can lead to performance issues if not managed properly. Using Promise.all
is a great way to handle multiple requests efficiently:
async function fetchMultipleData() {
let [data1, data2] = await Promise.all([
fetch('https://api.example.com/data1'),
fetch('https://api.example.com/data2')
]);
let result1 = await data1.json();
let result2 = await data2.json();
console.log(result1, result2);
}
2. Understanding the CORS Policy
Cross-Origin Resource Sharing (CORS) can be a stumbling block when working with APIs. CORS restrictions prevent requests to different domains unless the server explicitly allows it. To handle this, ensure your API server has the proper headers set or use a proxy server.
The Future of Async JavaScript and REST APIs
The demand for efficient, real-time applications continues to grow, and both async JavaScript and REST APIs will remain crucial components of modern web development. With emerging technologies like GraphQL and serverless architectures, the way we handle data requests is evolving, offering more flexibility and performance improvements.
Conclusion: Master Async JavaScript and REST APIs
Mastering async JavaScript and REST APIs is essential for any tech professional looking to build modern, efficient, and user-friendly applications. By understanding the core concepts and best practices, you can create seamless user experiences and enhance your coding capabilities.
Comments