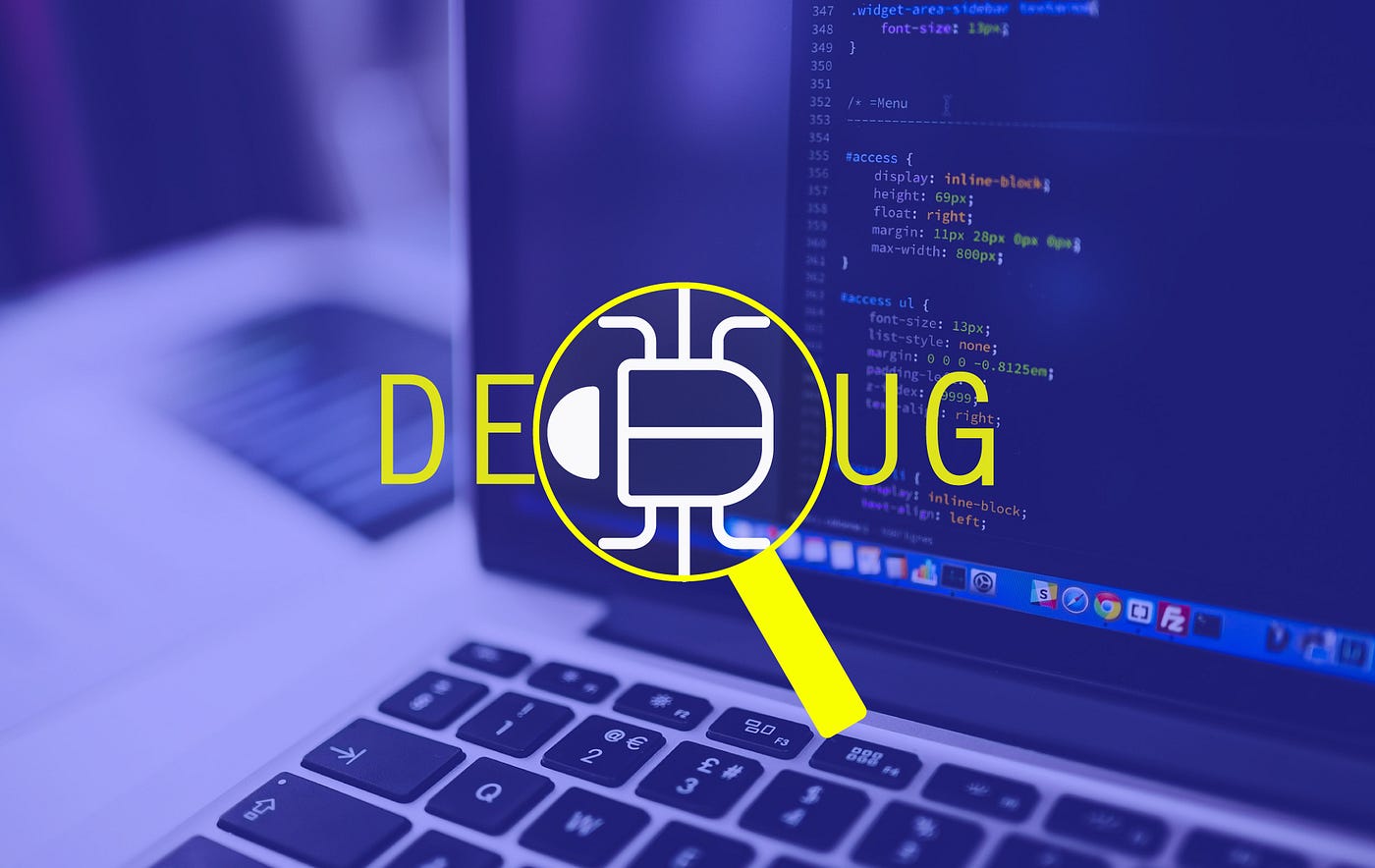
We've all been there—staring at our screen, trying to figure out why the code isn't working. That frustrating moment when you're sure everything should work, but it doesn’t. Coding bugs are inevitable, and while they can feel like the end of the world in the moment, fixing them doesn’t have to be a nightmare. Here’s a humane guide to debugging that’ll help you stay calm and productive.
1. Take a Deep Breath, It’s Part of the Process
Before you dive into the code, remind yourself that bugs are a natural part of coding. They don’t mean you’re a bad developer or that you’ve failed. In fact, finding and fixing bugs is what makes you a better developer. So, breathe, grab a coffee (or tea), and get ready to tackle the problem with a clear head.
2. Reproduce the Bug Consistently
One of the golden rules of debugging is being able to reproduce the bug. If you can’t reliably recreate the issue, it’s going to be hard to fix. Try to figure out exactly what steps lead to the problem. If it’s a user interface bug, interact with the app in the same way that caused the crash. If it’s a backend issue, retrace the API calls or database queries. Once you can reproduce it, you’re halfway there.
3. Break the Problem Down
Instead of looking at the entire codebase like an overwhelming mountain, break the problem into smaller chunks. Isolate the section of code where the issue is happening. This could mean narrowing it down to a specific function or loop. Trying to find a bug in 500 lines of code all at once is overwhelming, but working through 10 lines at a time? Much more manageable.
4. Add Logs and Print Statements
You may have heard people joke about "debugging by print statements," but guess what? It works. Adding simple print statements or logging can help you see what's happening at every stage of your code. Are variables changing when they shouldn't? Is a condition never being met? Logging gives you a window into the internal state of your program.
For example:
python
Copy code
print("User input:", user_input) print("Function output:", result)
Logs help you trace the flow of your program step-by-step. Don’t overuse them, but strategically placed logs can be a lifesaver.
5. Use a Debugger
If print statements aren’t cutting it, step things up with a proper debugger. Most modern IDEs (like VS Code, PyCharm, and Xcode) come with built-in debuggers that let you run your program line-by-line. You can set breakpoints, inspect variables, and even change values on the fly to see how they affect the outcome. It’s like having X-ray vision for your code.
6. Rubber Duck Debugging
This might sound silly, but talking out loud can actually help. “Rubber duck debugging” is the practice of explaining your code, line-by-line, to an inanimate object (or even to a colleague, if you prefer). By forcing yourself to articulate the problem, you often stumble upon the solution. It’s surprising how often a simple verbal explanation can reveal an oversight you didn’t notice while silently staring at the screen.
7. Google It (Seriously)
When you’ve exhausted all your ideas, don’t be afraid to Google the problem. Chances are, someone else has already run into the exact same issue. Stack Overflow is a goldmine of solutions. Just remember to be specific with your search—include error codes, programming language, and even version numbers. This will narrow down the results and save you from digging through pages of unrelated answers.
8. Ask for Help
When you’re stuck, it’s okay to ask for help. Whether it’s your colleague, a friend, or a coding community, sometimes a fresh set of eyes can make all the difference. But before asking, make sure you’ve done your homework—explain what the problem is, what you’ve tried, and any relevant logs or errors. People are much more willing to help when they see you’ve already made an effort.
9. Take a Break
If you’ve been staring at the code for hours and nothing makes sense, take a break. Go for a walk, grab a snack, or even sleep on it. It’s amazing how a little distance can give you a new perspective. Often, when you come back with fresh eyes, the solution will jump out at you. Burnout is real, and stepping away can help you return with a clear mind.
10. Learn from the Bug
Once you’ve fixed the bug (yes, you’ll get there!), take a moment to reflect. What caused the issue? Was it a misunderstanding of how a function worked? A logic error? Or something more obscure, like a race condition? Each bug is a learning opportunity. Write down what you learned, so you don’t make the same mistake twice.
Bonus: Automate Future Fixes
If the bug was caused by something simple—like an off-by-one error or a missing null check—consider adding tests to catch similar issues in the future. Writing unit tests or using static analysis tools can help prevent bugs from cropping up again. It might take a little extra time upfront, but it saves you a lot of headache down the road.
Comments