How to Fix "Uncaught TypeError: Cannot Read Property of Undefined" in JavaScript
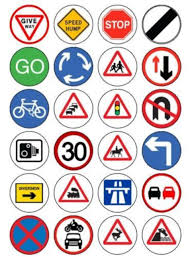
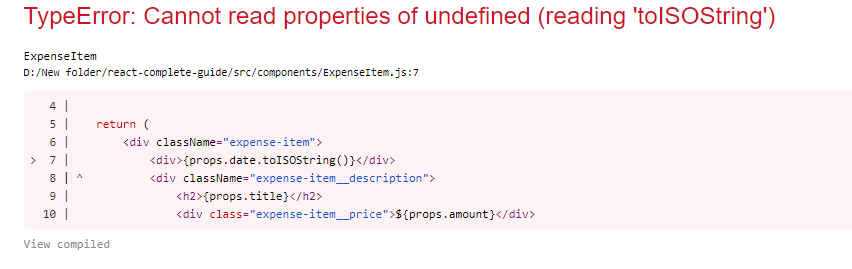
If you’ve ever worked with JavaScript, chances are you’ve come across the dreaded "Uncaught TypeError: Cannot read property of undefined" error. This is one of the most common errors you’ll encounter, and while it can be frustrating at first, the good news is that it’s usually pretty easy to fix—once you understand what's going on.
Let’s break it down and walk through how to solve this bug like a pro.
What Does This Error Mean?
When JavaScript tells you it "Cannot read property of undefined", it’s basically saying:
You're trying to access a property (like
name
,length
, orvalue
) of something that isundefined
.In simpler terms, JavaScript can’t find the object or variable you're asking it to read from.
For example, if you have this code:
let person; console.log(person.name);
Since person
is not defined (it's undefined
), JavaScript throws an error when you try to access person.name
.
Why Does This Happen?
This error commonly occurs for a few reasons:
Variables or Objects Are Not Properly Initialized
You’re trying to access a property of an object that hasn’t been created yet.API Data or Async Operations Return Undefined
You may be trying to work with data that hasn’t been loaded yet, such as when fetching data from an API.Misspelled or Incorrect Object References
You might accidentally reference a wrong object or key that doesn’t exist, or you’ve misspelled a property name.
Now, let's go through some practical ways to fix this error.
Step 1: Check if Your Variable is Initialized
The first thing you should do is check whether the variable or object you’re trying to access actually exists. A simple way to prevent the error is to use optional chaining (if you're using ES2020 and beyond) or conditionals.
Here’s an example using optional chaining:
let person = {}; console.log(person?.name); // This will return undefined, but not throw an error
The ?.
checks if person
exists before trying to access the name
property. If person
is undefined
or null
, it will simply return undefined
instead of crashing your code.
If you're not using optional chaining, you can do this the old-school way:
let person = {}; if (person && person.name) { console.log(person.name); } else { console.log('Name property is not available'); }
Step 2: Ensure Your Data is Loaded Before Accessing It
If you’re working with data fetched from an API or some other asynchronous operation, there’s a chance your data isn’t available yet when you try to access it. JavaScript might be trying to read the property before the data is fully loaded, causing the bug.
Here’s how to avoid that issue:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Check if the data exists before accessing it if (data && data.user) { console.log(data.user.name); } else { console.log('User data is not available'); } }) .catch(error => console.error('Error fetching data:', error));
This ensures that you only try to access data.user.name
if data.user
is actually defined.
Step 3: Handle Nested Objects Safely
Sometimes, the error happens when you're trying to access deeply nested properties. If any level of the object is undefined
, the error will occur. For example:
let data = {}; console.log(data.user.name); // Uncaught TypeError: Cannot read property 'name' of undefined
To avoid this, you can use optional chaining again:
let data = {}; console.log(data?.user?.name); // Safely returns undefined without crashing
Or handle it with more verbose checks:
if (data && data.user && data.user.name) { console.log(data.user.name); } else { console.log('User or name is undefined'); }
Step 4: Debugging with Console Logs
If you're unsure where the error is coming from, the best debugging tool is console.log
. Add logs at key points in your code to inspect the variables you're working with:
let person = getPersonData(); console.log(person); // This will show you what person looks like console.log(person.name); // And whether person.name is actually defined
By logging the variables, you can easily trace where things are going wrong and fix the issue.
Step 5: Use Try-Catch for Critical Operations
If you’re working with critical code and you want to ensure the program keeps running even when an error occurs, wrap your code in a try-catch
block:
try { let person = getPersonData(); console.log(person.name); } catch (error) { console.error('Error:', error.message); }
This won’t prevent the error, but it will allow your program to continue running gracefully instead of crashing entirely.
Common Use Cases and Fixes
Undefined API Data: If you're working with data from an external API, always check if the data has been loaded before trying to access properties.
Missing DOM Elements: When manipulating the DOM, ensure that the element you’re trying to reference exists on the page:
let button = document.getElementById('submit-btn'); if (button) { button.addEventListener('click', handleSubmit); } else { console.error('Submit button not found'); }
Misconfigured State in React/Vue: In front-end frameworks, you often deal with state that might not be immediately available. Use conditionals to check whether the state has been properly set before rendering components.
The "Cannot read property of undefined" error can feel annoying, but it’s really just JavaScript’s way of saying "Hey, I can’t find what you’re looking for!" By understanding the common causes and using tools like optional chaining, conditional checks, and logging, you can easily fix this error and avoid it in the future.Remember, bugs are just part of the coding journey. Each one you solve helps you grow as a developer, and soon enough, fixing them will become second nature. Happy coding! :)
Comments