Can I Use React Query with Next.js? A Comprehensive Guide for Developers
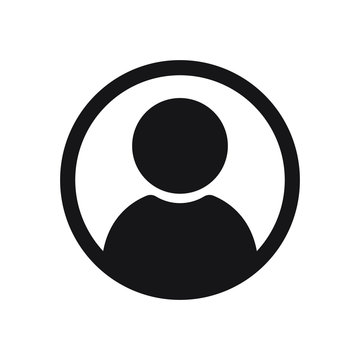
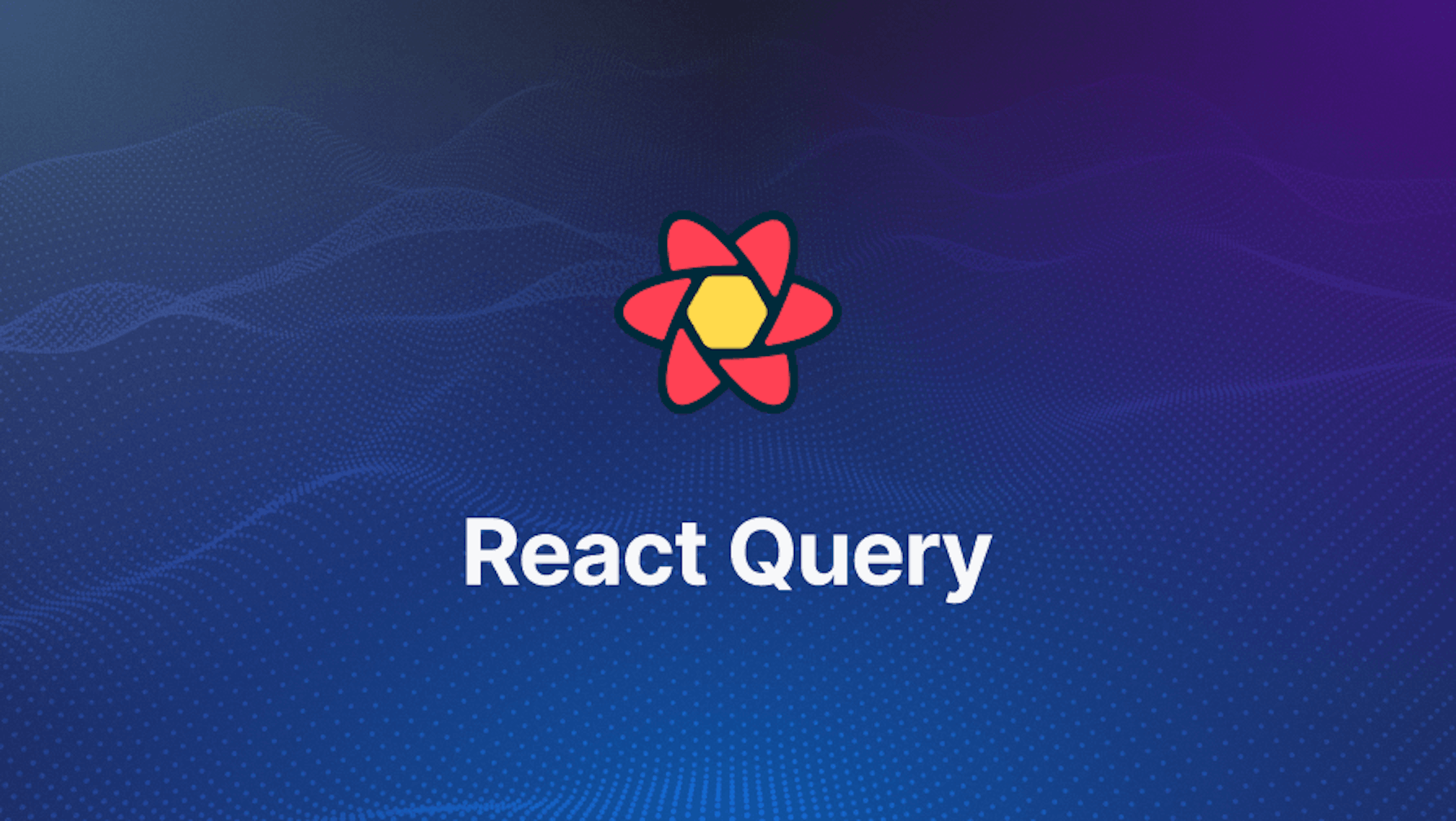
As a developer, you’re likely familiar with Next.js and its powerful features for server-side rendering (SSR) and static site generation (SSG). But what happens when you need to fetch, cache, and synchronize remote data seamlessly in your Next.js application? Enter React Query, a game-changer for handling server state. But can you use React Query with Next.js effectively? The short answer is yes, and in this article, we’ll explore exactly how to do that.
Why Use React Query with Next.js?
Before diving into how to use React Query in Next.js, let’s start with why you should consider combining these two popular libraries.
React Query is a library for managing server state in React applications. It simplifies tasks such as fetching, caching, synchronizing, and updating remote data while providing hooks that allow you to handle asynchronous requests effortlessly.
Next.js, on the other hand, is a React framework designed to build fast, production-ready applications using SSR and SSG. Its focus on performance and SEO makes it an ideal candidate for modern web applications.
Using React Query in a Next.js environment offers several benefits:
Optimized Data Fetching: React Query’s cache-first approach ensures efficient data fetching.
Built-in Caching: The library automatically caches and updates data, eliminating unnecessary requests.
Improved Developer Experience: Say goodbye to manually managing loading states or errors, as React Query handles it all.
Setting Up React Query in Next.js
Let’s walk through the process of setting up React Query with Next.js.
1. Install React Query
If you’re using TypeScript (which I highly recommend for Next.js apps)
npm install @tanstack/react-query
2. Create a Query Client
React Query uses a QueryClient to manage the cache and fetch data. You need to create an instance of QueryClient
and wrap your application with the QueryClientProvider
.
In your Page.tsx
at your app folder, wrap your app with the React Query provider:
// _app.js
import { QueryClient, QueryClientProvider } from 'react-query';
const queryClient = new QueryClient();
function MyApp({ Component, pageProps }) {
return (
<QueryClientProvider client={queryClient}>
<Component {...pageProps} />
</QueryClientProvider>
);
}
export default MyApp;
3. Fetch Data Using React Query
Now, let’s use the useQuery
hook to fetch data. React Query abstracts away the complexity of managing data fetching, loading states, and error handling. Here’s an example of how you can fetch user data from an API:
import { useQuery } from 'react-query';
import axios from 'axios';
function fetchUsers() {
return axios.get('/api/users');
}
export default function Users() {
const { data, error, isLoading } = useQuery('users', fetchUsers);
if (isLoading) return <p>Loading...</p>;
if (error) return <p>Error loading data</p>;
return (
<div>
{data.map((user) => (
<div key={user.id}>{user.name}</div>
))}
</div>
);
}
React Query makes handling data fetching a breeze, and you no longer need to manage loading states manually.
Benefits of Using React Query in Next.js
The combination of React Query and Next.js is powerful, especially when working with server-side data fetching. Here’s why:
1. Server-Side Rendering (SSR) with React Query
SSR can be a bit tricky when working with React Query because the server needs to pre-fetch the data before rendering. Luckily, React Query provides a way to use SSR by hydrating the data on the server.
In Next.js, you can use the getServerSideProps
function to fetch data on the server, then hydrate the cache on the client:
export async function getServerSideProps() {
const queryClient = new QueryClient();
await queryClient.prefetchQuery('users', fetchUsers);
return {
props: {
dehydratedState: dehydrate(queryClient),
},
};
}
This ensures that your app is SEO-friendly and provides a smooth user experience by rendering the content on the server.
2. Automatic Data Refetching
One of the standout features of React Query is its ability to automatically refetch data when necessary. Whether the user navigates away and returns to your app or performs a mutation that updates the data, React Query will intelligently refetch and sync the data.
This is especially useful in a Next.js environment, where performance is key.
3. Optimistic Updates
When dealing with user actions like form submissions, React Query supports optimistic updates, allowing you to instantly update the UI without waiting for the server response. Once the server confirms the update, React Query refines the data, ensuring a seamless experience for the user.
Common Questions About Using React Query in Next.js
1. How does React Query compare to SWR?
Both React Query and SWR (stale-while-revalidate) are excellent data-fetching libraries, but they serve different purposes. SWR focuses on simplicity and client-side caching, while React Query is more feature-rich, offering server-side caching, mutations, and fine-tuned control over data synchronization.
2. Can I use React Query with static site generation (SSG)?
Yes, you can! React Query works with SSG as well, but there’s a caveat. With SSG, data is pre-fetched at build time, so you won’t get the same automatic updates React Query provides in SSR. However, for most use cases, SSG with React Query is a great combination for building static sites with dynamic data.
A Personal Experience Using React Query in a Next.js Project
In one of my projects, I was building a dashboard where users could view and manage tasks. Initially, I was handling data fetching with useEffect
, but the code quickly became unmanageable with the need for manual cache management and error handling.
Switching to React Query with Next.js solved all these problems. With built-in caching, automatic refetching, and simplified code, my app became faster and more efficient. I could focus more on building features instead of managing API calls.
Mastering Async JavaScript and REST APIs with React Query
To take full advantage of React Query, it’s essential to have a solid grasp of Async JavaScript and REST APIs. By mastering async JavaScript, you’ll better understand how React Query handles promises, making your coding experience even smoother.
If you’re dealing with API errors and find yourself frustrated, check out our guide on How to Fix Coding Bugs Without Losing Your Mind. Debugging can be tough, but with the right tools and mindset, you’ll resolve issues in no time.
Conclusion: Should You Use React Query with Next.js?
Absolutely. React Query and Next.js complement each other beautifully, allowing you to build high-performance applications with ease. Whether you’re working on SSR, SSG, or client-side rendering, React Query’s data management capabilities will enhance your development workflow.
So, are you ready to start using React Query in your Next.js projects? What challenges have you faced with data fetching in Next.js, and how do you plan to overcome them? Let us know in the comments!
Comments